Vue 애플리케이션 기초 가이드
선언적 렌더링
템플릿(template) 구문을 사용하여 DOM에 데이터(data)를 렌더링하는 선언적 렌더링에 대해 학습해 봅니다.
<template>
<div id="app">
<img class="logo" src="./assets/logo.png" alt="Vue.js">
<p><strong>{{ declarative_rendering }}</strong></p>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
declarative_rendering: '선언적 렌더링'
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
속성 바인딩
v-bind
디렉티브를 사용해 데이터를 DOM 속성으로 연결하는 방법을 학습해 봅니다.
<template>
<div id="app">
<p>우리
<time
v-bind:datetime="now"
v-bind:title="now"
>지금</time>
당장 만나!
</p>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
now: new Date().toISOString()
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
NOTE
toISOString() 메서드는 ISO 형식(ISO 8601)의 문자열을 반환합니다. 반환값은 언제나 24글자 또는 27글자(각각 YYYY-MM-DDTHH:mm:ss.sssZ
또는 ±YYYYYY-MM-DDTHH:mm:ss.sssZ
)입니다. 시간대는 언제나 UTC이며 접미어 "Z"로 표현합니다.
조건부 렌더링
v-if
디렉티브를 사용하여 DOM에 조건부 렌더링하는 방법을 학습해 봅니다.
<template>
<div id="app">
<p v-if="is_visible">{{ declarative_rendering }}</p>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
is_visible: true,
declarative_rendering: '조건부 렌더링'
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
리스트 렌더링
v-for
디렉티브를 사용해 데이터(예: 배열)를 순환하여 DOM에 리스트 렌더링하는 학습해 봅니다.
<template>
<div id="app">
<ul>
<li
v-for="(todo, i) of todos"
v-bind:key="i"
>
{{ todo.it }}
</li>
</ul>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
todos: [
{ it: 'Vue.js 학습' },
{ it: 'Nuxt.js 학습' },
]
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
이벤트 바인딩
v-on
디렉티브를 통해 이벤트 바인딩된 컴포넌트 메서드를 실행하여 데이터를 수정, UI를 업데이트 하는 학습을 합니다.
<template>
<div id="app">
<button type="button" v-on:click="addStar">스타 추가</button>
<p>{{ star_message }}</p>
</div>
</template>
<script>
export default {
name: "App",
data: () => ({
star_message: 'v-on 이벤트 연결'
}),
methods: {
addStar() {
this.star_message = `
${'*'.repeat(2)} ${this.star_message} ${'*'.repeat(2)}
`;
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
컴포넌트
컴포넌트에서 다른 컴포넌트를 불러와 등록하고 사용하는 방법을 학습합니다. (컴포넌트 ≓ 커스텀 태그)
App.vue
<template>
<div id="app">
<add-star-button/>
<p>{{ star_message }}</p>
</div>
</template>
<script>
import AddStarButton from './components/AddStarButton';
export default {
name: "App",
components: { AddStarButton },
data: () => ({
star_message: '컴포넌트'
})
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
components/AddStarButton.vue
<template>
<button type="button" v-on:click="addStar">
스타 추가
</button>
</template>
<script>
export default {
name: 'AddStarButton',
methods: {
addStar() {
let msg = this.$parent.star_message;
if (msg) {
this.$parent.star_message = `
${'*'.repeat(2)} ${msg} ${'*'.repeat(2)}
`;
}
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
글로벌 컴포넌트
별도의 컴포넌트 호출/등록 과정 없이 모든 컴포넌트에서 컴포넌트를 사용하기 위해 글로벌 컴포넌트로 등록하는 방법을 학습합니다.
main.js
import Vue from 'vue';
// 글로벌 컴포넌트 등록
import AddStarButton from './components/AddStarButton';
Vue.component('add-star-button', AddStarButton);
2
3
4
5
components/App.vue
<template>
<div id="app">
<add-star-button/>
<p>{{ star_message }}</p>
</div>
</template>
<script>
export default {
name: "App",
data: () => ({
star_message: '글로벌 컴포넌트 활용'
})
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
슬롯
컴포넌트 내부로 콘텐츠를 삽입하거나, 다른 컴포넌트를 중첩하기 위한 슬롯(Slot) 사용법을 학습합니다.
App.vue
<add-star-button>별 추가</add-star-button>
components/AddStarButton.vue
<template>
<button type="button" v-on:click="addStar">
<slot>스타 추가</slot> <!-- 내부에 포함된 값은 슬롯의 기본 값입니다. -->
</button>
</template>
2
3
4
5
NOTE
컴퓨터의 램 슬롯을 떠올려 보면, 슬롯이 가지는 "끼워넣다"는 의미가 보다 쉽게 이해될 겁니다.
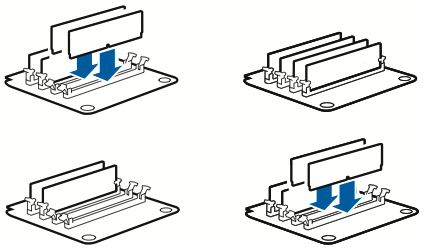
웹 컴포넌트와 비교
Vue 컴포넌트는 W3C 웹 컴포넌트 기술과 유사하게 구현되었습니다. 웹 컴포넌트를 정리한 문서와 예제를 참고하세요.
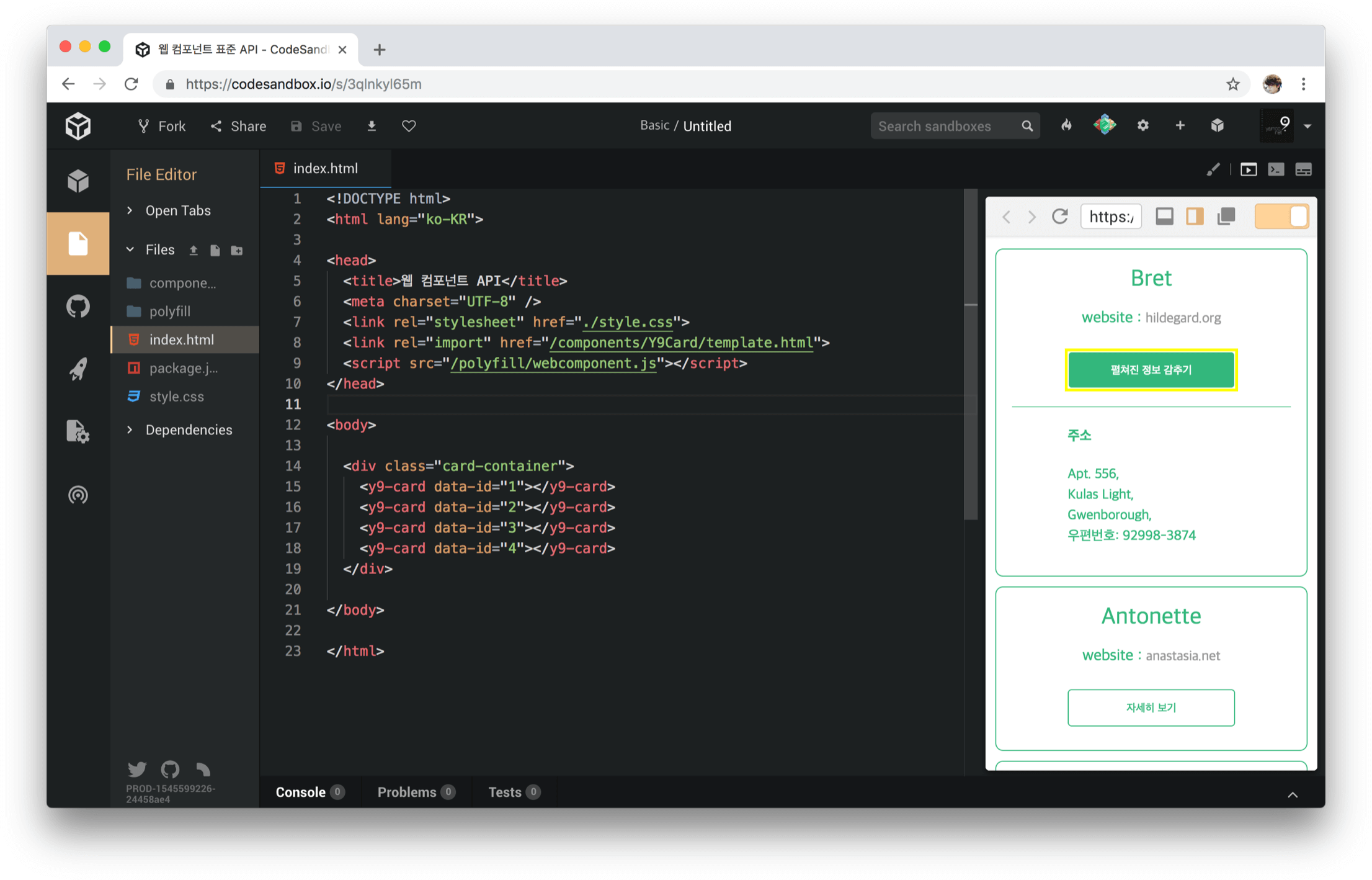
TIP
Vue.js를 사용해 인스턴트 프로토타이핑 개발 후, 웹 컴포넌트로 배포하는 방법을 사용하면 보다 손쉽습니다.
컴포넌트 통신
컴포넌트 외부로 사용자가 정의한 이벤트를 요청하고자 한다면 $emit() 메서드를 사용해 이벤트 이름을 정의하고, 전달할 인자를 필요한 만큼 추가해 페이로드(Payload)를 전송할 수 있습니다.
components/AddStarButton.vue
<template>
<!-- 버튼 클릭 시, 이벤트 송신 요청 -->
<button type="button" v-on:click="addStar">
<slot>스타 추가</slot>
</button>
</template>
<script>
export default {
name: 'AddStarButton',
methods: {
addStar() {
// 이벤트 송신
this.$emit('onAddStar', '스타', '추가', '별(*)');
}
}
}
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
App.vue
<template>
<div id="app">
<!-- 이벤스 수신 -->
<add-star-button v-on:onAddStar="addStar">
별 추가
</add-star-button>
<p>{{ star_message }}</p>
</div>
</template>
<script>
import AddStarButton from './components/AddStarButton';
export default {
name: "App",
components: { AddStarButton },
data: () => ({
star_message: '컴포넌트 이벤트 수신'
}),
methods: {
// 이벤트가 수신되면 실행되는 컴포넌트 메서드
addStar() {
this.star_message = `${'*'.repeat(2)} ${this.star_message} ${'*'.repeat(2)}`
}
}
};
</script>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
← Vue 애플리케이션 특징 Vue 객체 →