Generator
Generator 객체는 제너레이터 함수(function* () {}
)로부터 반환된 값이며 이터레이션 프로토콜을 준수합니다.
영상 강의
PART 1
예제
피보나치의 수를 생성하는 함수
피보나치의 수를 생성하는 fibonacci
제너레이터 함수는 다음과 같이 작성합니다.
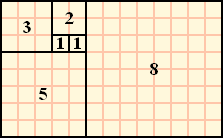
function* fibonacci(n=1) {
let [ current, next ] = [1, 1];
while(n--) {
yield current;
[ current, next ] = [ next, current + next ];
}
}
let fibo10 = fibonacci(10);
[...fibo10]; // [1, 1, 2, 3, 5, 8, 13, 21, 34, 55]
간단한 ID 생성 함수
간단한 ID를 생성하는 제너레이터 함수 idMaker
는 다음과 같이 작성할 수 있습니다.
function* idMaker(id=10000, prefix="id") {
while(true) {
yield `${prefix}-${Math.floor(Math.random() * id)}`;
}
}
const ids = idMaker();
ids.next().value; // "id-743"
ids.next().value; // "id-985"
ids.next().value; // "id-5198"
고유키 생성 함수
고유키를 생산하는 제너레이터 함수 uniqueIdMaker
는 다음과 같이 작성할 수 있습니다.
function* uniqueIdMaker(count=5, limit=10) {
const keys = 'abcdefghijklmnopqrstuvwxyz!@#1234567890'.split('');
function _uid(count){
let randomKey = '';
while(count--) {
randomKey += keys[Math.floor(Math.random() * keys.length)];
}
return randomKey;
}
while(limit--) {
yield _uid(count);
}
}
const uid = uniqueIdMaker(10, 3);
console.log([...uid]); // ["1rk#8p57ji", "#qwhk6wuwx", "wg5fc06i0e"]
참고
← Iteration Object Set →